One important feature in the field of electronic and embedded technology that finds use in energy management, interactive environments, and security systems is the ability to identify and respond to human presence and movements.
Processing and interpreting the information with a mix of sensors and microcontrollers is one efficient approach to accomplish this.
The STHS34PF80 sensor, an Arduino, IC 7809 and a few other parts like resistors and capacitors are used in this tutorial to create a simple yet functional circuit.
The Arduino microcontroller will function as the circuits central processing unit, reading sensor data and applying the appropriate logic to it.
The STHS34PF80 is a capacitive proximity sensor that uses variations in capacitance to identify activity or the existence of objects close by.
This sensor is very helpful in situations that call for contactless sensing.
It is perfect for use in a range of motion sensing applications since it is sensitive to the presence of human bodies and can detect motion through fluctuations in capacitance.
Code with Explanation:
#include <Arduino.h>
const int sensorPin = A0;
const int threshold = 500; // Adjust threshold as needed
void setup() {
Serial.begin(9600);
}
void loop() {
int sensorValue = analogRead(sensorPin);
if (sensorValue > threshold) {
Serial.println("Human detected!");
} else {
Serial.println("No human detected");
}
delay(100); // Adjust delay as needed
}
Explanation:
- The header file required for Arduino programming is included with the #include line, giving access to a number of functions and libraries.
- Two constants are defined by the lines const int threshold = 500; and const int sensorPin = A0;:
- SensorPin represents the Arduino analog input pin (A0 in this example) to which the sensor is attached.
- Threshold describes the value at which a human can be detected as present or moving.
- The sensor readout shows human presence if it surpasses this level.
- In order to enable data printing to the serial monitor for debugging and monitoring, Serial.begin(9600); initializes the serial communication at a baud rate of 9600.
- Loop Purpose: reads the analog value from the sensor pin and puts it in the sensorValue variable (int sensorValue = analogRead(sensorPin)).
- The code snippet if (sensorValue > threshold) {… } else {… } determines whether the sensorValue exceeds the threshold.
- If so, the serial monitor prints the message “Human detected!” indicating the presence of a human.
- If not, “No human detected” appears on the screen.
- delay(100); creates a 100 ms delay prior to the loop’s repetition, enabling a brief break in between measurements.
Circuit Working:
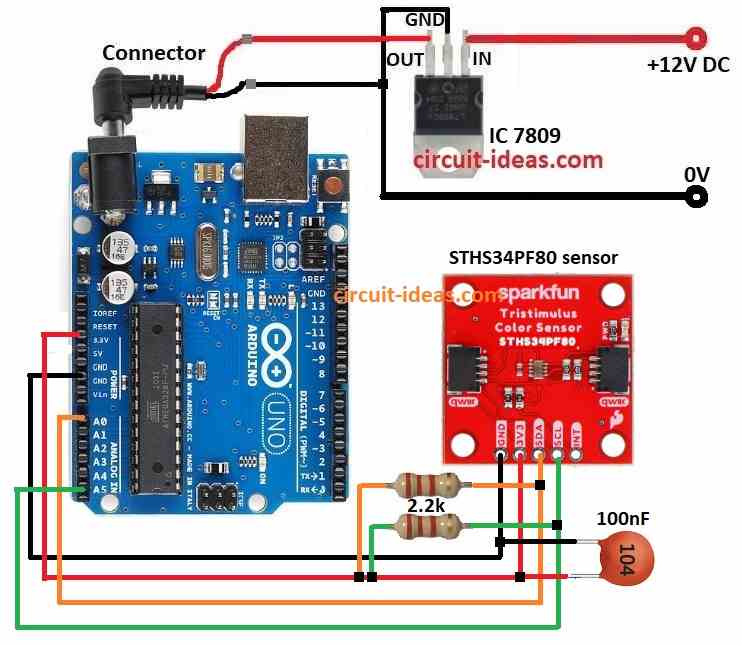
Parts List:
Component | Quantity |
---|---|
Arduino Uno Board | 1 |
IC 7809 | 1 |
STHS34PF80 Human Sensor | 1 |
Capacitor 100nF | 1 |
Resistors 2.2k, 1/4 watt | 2 |
In this article we have included the IC 7809 to provide a steady and controlled power supply for the Arduino and STHS34PF80 sensor.
The Arduino and sensor will operate dependably since the IC 7809 outputs a steady 9V.
It helps in the reduction of noise and voltage variations that might skew sensor data.
The circuit components can be shielded from harm by the IC 7809 from overvoltage or overcurrent.
The STHS34PF80 is a capacitive sensor that measures temperature and humidity.
Still, we mainly concentrate on its capacity to sense variations in the capacitive field around it in our application.
The capacitance of the surrounding environment varies as a human enters or travels within the sensors range.
Signal conditioning is required before the Arduino can process the raw analog signal that comes from the sensor.
The circuits resistors and capacitor work together as a low-pass filter to smooth out high-frequency noise and smooth down the raw data.
The sensors filtered analog signal is read by the Arduino.
A humans presence or mobility can be detected by the microcontroller by comparing the current reading to a threshold value.
A person is present if the reading is higher than the threshold.
How to Build:
To build a Human Presence and Motion Sensor Circuit using Arduino follow the below mentioned steps for connections:
- Gather all the components as mentioned in the above circuit diagram.
- Connect a regulated IC1 7809 to provide a regulated 9V DC to the Arduino board as per the above circuit diagram
- Connect STHS34PF80 sensor GND pin to GND pin on Arduino.
- Connect STHS34PF80 sensor 3.3V pin to 3.3V pin on Arduino.
- Connect STHS34PF80 sensor SDA pin to A0 on Arduino
- Connect STHS34PF80 sensor SCL pin to A5 on Arduino
- Connect capacitor 100nF from positive of 3.3V to ground.
- Connect one end of 2.2k resistor form STHS34PF80 sensor SDA pin and other end to 3.3V positive supply.
- Connect one end of other 2.2k resistor to STHS34PF80 sensor SCL pin and other other end to 3.3V positive supply.
Conclusion:
The STHS34PF80 sensor and Arduino are used in the Human Presence and Motion Sensor Circuit to give a basic and efficient method of detecting motion and human presence.
Through tweaking the threshold value and additional factors, the circuit may be tailored to fit certain uses.
Numerous projects, including interactive installations, security systems, and home automation, can make use of this circuit.
Leave a Reply