For many applications, including industrial automation, safety monitoring and fire alarm systems, flame sensor is crucial.
In order to detect fire or flames, we will examine in this post how to connect a flame sensor to an Arduino.
This project offers an affordable yet efficient method for using flame detection in practical situations.
Circuit Working with Code:
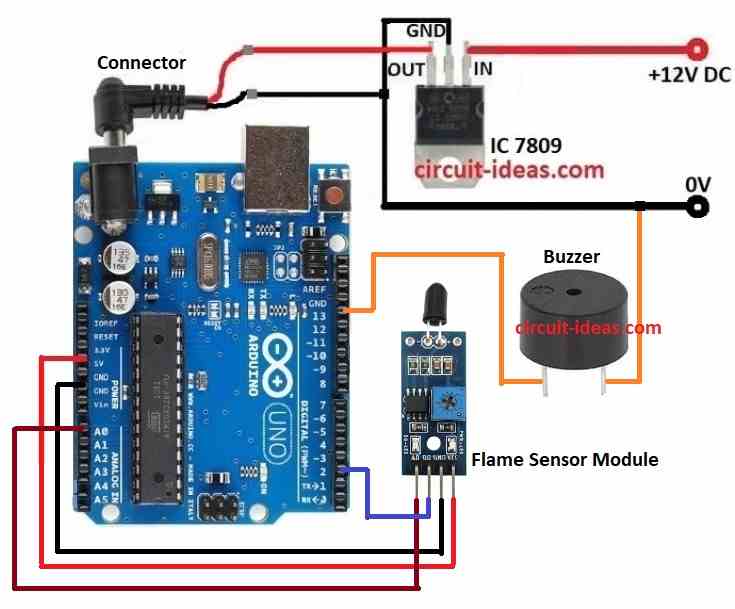
Parts List:
Component | Quantity |
---|---|
IC 7809 | 1 |
Arduino Uno | 1 |
Flame Sensor Module 4 pin | 1 |
Buzzer | 1 |
Coding for Flame Sensor Digital (D0) Output:
// Pin Definitions
const int BUZZER_PIN = 13; // Pin connected to the buzzer
const int FLAME_SENSOR_PIN = 2; // Pin connected to the flame sensor
void setup() {
// Set pin modes
pinMode(BUZZER_PIN, OUTPUT);
pinMode(FLAME_SENSOR_PIN, INPUT);
// Initialize serial communication
Serial.begin(9600);
}
void loop() {
// Read the flame sensor value
int flameDetected = digitalRead(FLAME_SENSOR_PIN);
// Check if flame is detected
if (flameDetected == HIGH) {
Serial.println("Flame Detected!");
digitalWrite(BUZZER_PIN, HIGH); // Turn on the buzzer
} else {
Serial.println("No Flame");
digitalWrite(BUZZER_PIN, LOW); // Turn off the buzzer
}
// Add a small delay for stability
delay(100);
}
Coding for Flame Sensor Analog (A0) Output:
// Pin Definitions
const int ANALOG_PIN = A0; // Flame sensor connected to analog input pin A0
const int BUZZER_PIN = 13; // Buzzer connected to pin 13
const int THRESHOLD = 400; // Flame detection threshold
void setup() {
pinMode(BUZZER_PIN, OUTPUT); // Set the buzzer pin as an output
Serial.begin(9600); // Initialize serial communication
}
void loop() {
// Read the value from the flame sensor
int analogValue = analogRead(ANALOG_PIN);
Serial.print("Flame Sensor Value: ");
Serial.println(analogValue); // Print the sensor value for debugging
// Check flame levels
if (analogValue > THRESHOLD) {
digitalWrite(BUZZER_PIN, HIGH); // Activate buzzer
Serial.println("High Flame Detected!");
}
else if (analogValue == THRESHOLD) {
digitalWrite(BUZZER_PIN, HIGH); // Activate buzzer briefly
Serial.println("Low Flame Detected!");
delay(400); // Short delay to indicate low flame
digitalWrite(BUZZER_PIN, LOW); // Deactivate buzzer
}
else {
digitalWrite(BUZZER_PIN, LOW); // Deactivate buzzer
Serial.println("No Flame Detected.");
}
// Small delay to stabilize sensor readings
delay(100);
}
In this post the input voltage is controlled to a steady 9V using the IC 7809.
If your power source offers a greater or varying voltage, like 12V from a battery or an unregulated power supply this is helpful.
It guarantees a steady voltage for your parts, such as the Arduino and flame sensor (using a step-down circuit).
The flame sensor device picks up infrared light from flames and produces an analog voltage that is proportionate to the lights intensity.
Stronger flame intensity is indicated by a greater analog value.
Additionally, a digital output pin on the sensor toggles between HIGH and LOW based on whether the flame intensity surpasses a predetermined threshold.
The Arduino reads the sensors output continually.
The buzzer is triggered to notify the user if the flame intensity exceeds the threshold.
For logging or debugging reasons, the system can additionally show the flame intensity on the Serial Monitor.
Upon detection of the flame, the buzzer acting as a warning signal is triggered.
Applications:
- Fire alarm systems are used in homes and businesses to detect and warn of fires.
- Keep an eye out for fire risks in potentially dangerous places, such as laboratories or kitchens.
- Make sure that procedures that use fire, such kilns or furnaces are safe.
Formula:
Analog-to-Digital Conversion (ADC):
The Arduino utilizes its integrated ADC to change the analog voltage from the flame sensor into a digital value.
The equation for the transformation is:
Vin = (ADC Value × Vref) / 1023
where,
- Vin is the voltage from the flame sensor
- ADC Value is the digital value from analogRead() (0 to 1023)
- Vref is reference voltage (usually 5V)
You can use this formula to map the sensors analog output to a voltage level.
How to Build:
To build a Simple Flame Sensor Circuit using Arduino follow the below mentioned steps for connections:
- Gather all the components as mentioned in the above circuit diagram.
- Connect a regulated IC 7809 to provide a regulated 9V DC to the Arduino board as per the above circuit diagram
- Connect the analog output A0 pin of the flame sensor to the Arduinos analog input pin A0.
- Connect the digital output D0 to pin 2 of digital pin on the Arduino.
- Connect the VCC and GND pins of the flame sensor module to the 5V and GND of the Arduino.
- Connect the positive terminal of the buzzer to Arduino pin 13 and connect the negative terminal to GND.
Conclusion:
The Arduino flame sensor circuit is a basic yet efficient flame detection project.
An Arduino, a buzzer and a flame sensor may be used to make a dependable flame detection system for a range of uses.
This project is an outstanding example of how simple sensors may be connected to microcontrollers to address practical issues.
Leave a Reply