This project shows how to regulate the speed of a DC motor with an Arduino microcontroller, a MOSFET transistor, and a potentiometer.
The Arduino creates a Pulse Width Modulation PWM signal that controls the MOSFETs gate.
The MOSFET acts as a switch, regulating the voltage provided to the motor and so managing its speed.
The Arduino DC Motor Speed Controller is a simple and cheap tool that can control motors, lights, and heaters.
You can easily adjust its speed and power.
Programming Code:
const int motorPin = 9; // PWM pin for motor control
const int potPin = A0;
void setup() {
pinMode(motorPin, OUTPUT);
}
void loop() {
int potValue = analogRead(potPin);
int motorSpeed = map(potValue, 0, 1023, 0, 255);
analogWrite(motorPin, motorSpeed);
}
Code Explanation:
- Motor pin defines the Arduino pin that connects to the MOSFET gate.
- Potentiometer pin defines the Arduino analog pin that connects to the potentiometer.
- Setup() initializes the motor pin as an output.
- loop() reads the potentiometer value continuously, converts it to a motor speed value between 0 and 255, and configures the motor pins PWM duty cycle.
Circuit Working:
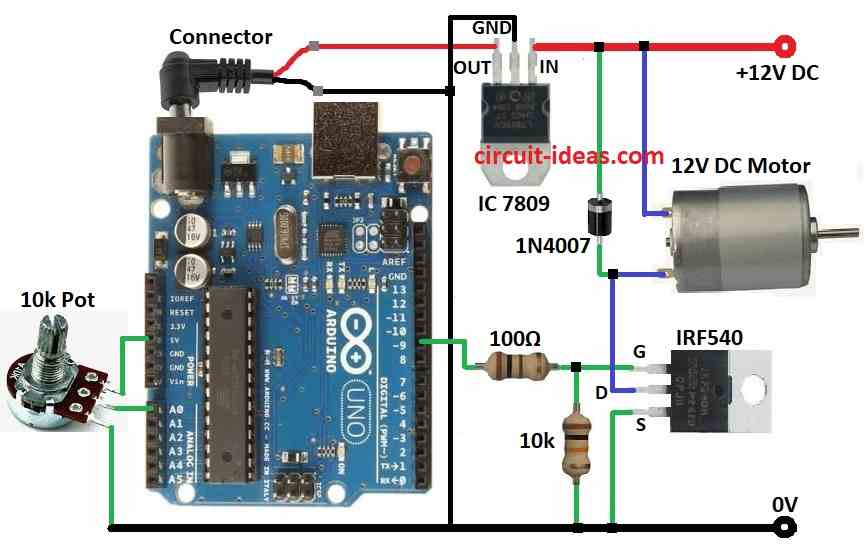
Parts List:
Component | Quantity |
---|---|
Arduino UNO | 1 |
IC 7809 | 1 |
MOSFET IRF540 | 1 |
Resistor (1/4 watt 100Ω) | 1 |
Resistor (1/4 watt 10k) | 1 |
Potentiometer 10k | 1 |
Diode 1N4007 | 1 |
DC Motor 12V 1Amp | 1 |
In this article 12V power source powers the circuit.
The Arduino creates the PWM signal that controls the MOSFET.
10k potentiometer determines the duty cycle of the PWM signal, which controls motor speed.
The MOSFET functions as a switch, controlled by the PWM signal.
It adjusts the voltage applied to the DC motor.
A diode is connected in parallel with the motor to avoid the reverse EMF generated by the motor coil.
The motor comes with its own 12V power source.
The voltage provided through the MOSFET controls the motors speed.
How to Build:
To build a DC Motor Speed Controller Circuit using Arduino following are the connections steps to follow:
- Gather all the required components as mentioned in the above circuit diagram
- Connect the Arduinos 5V and ground pins to the PCB.
- Connect the potentiometers one outer pin to the 5V, the middle pin to analog pin A0 of the Arduino board and connect the other outer pin of the potentiometer to ground
- Connect the 100 ohm resistor between the Arduinos digital pin 9 and the gate of the MOSFET.
- Connect 10k resistor between gate and ground of MOSFET.
- Connect the source of the MOSFET to the ground.
- Connect the drain of the MOSFET to the one terminal of the motor.
- Connect the other terminal of the motor to the positive supply of 12V.
- Connect the diode in reverse bias across the motor terminals.
- Connect a regulated IC 7809 to provide a regulated 9V DC to the Arduino board.
Conclusion:
By constructing a DC motor speed control system using an Arduino, a MOSFET and a potentiometer.
The Arduino generates the PWM signal, the MOSFET controls the motor voltage, and the potentiometer allows for variable speed control.
This project uses electrical components and microcontrollers to explain essential motor control principles.
Leave a Reply