Developing visually attractive projects may greatly improve learning and engagement in the field of electronics.
Circle Running LED is one such project that uses an Arduino microcontroller to operate a series of LEDs placed in a circle.
This project is not only simple to make but also gives insights into programming, circuit design and LED control.
A 10k potentiometer allows users to modify the LED sequences pace, giving the display an interactive component.
Code with Explanation:
Arduino Pin 2 ----|120Ω|----|>| LED1
Arduino Pin 3 ----|120Ω|----|>| LED2
...
Arduino Pin 9 ----|120Ω|----|>| LED8
A0 ------|------- Potentiometer -------|--- 5V
|--- GND (other pin)
|---- 7809 ---- Power rail for LEDs
const int ledPins[] = {2, 3, 4, 5, 6, 7, 8, 9}; // Define LED pins
const int potPin = A0; // Define potentiometer pin
int potValue; // Variable to store potentiometer value
int delayTime; // Variable to control delay based on pot value
void setup() {
// Initialize LED pins as outputs
for (int i = 0; i < 8; i++) {
pinMode(ledPins[i], OUTPUT);
}
}
void loop() {
potValue = analogRead(potPin); // Read the potentiometer value (0-1023)
delayTime = map(potValue, 0, 1023, 50, 500); // Map it to a delay range (50ms to 500ms)
for (int i = 0; i < 8; i++) {
digitalWrite(ledPins[i], HIGH); // Turn on the current LED
delay(delayTime); // Wait for a duration based on potentiometer
digitalWrite(ledPins[i], LOW); // Turn off the current LED
}
}
Explanation:
- The pin numbers assigned to the LEDs are stored in the ledPins array.
- Every LED pin is assigned to be an output by the setup() method.
- The loop() function adds a delay decided by the potentiometer to each LED turn-on sequence while constantly reading the potentiometer value and mapping it to a delay duration.
Circuit Working:
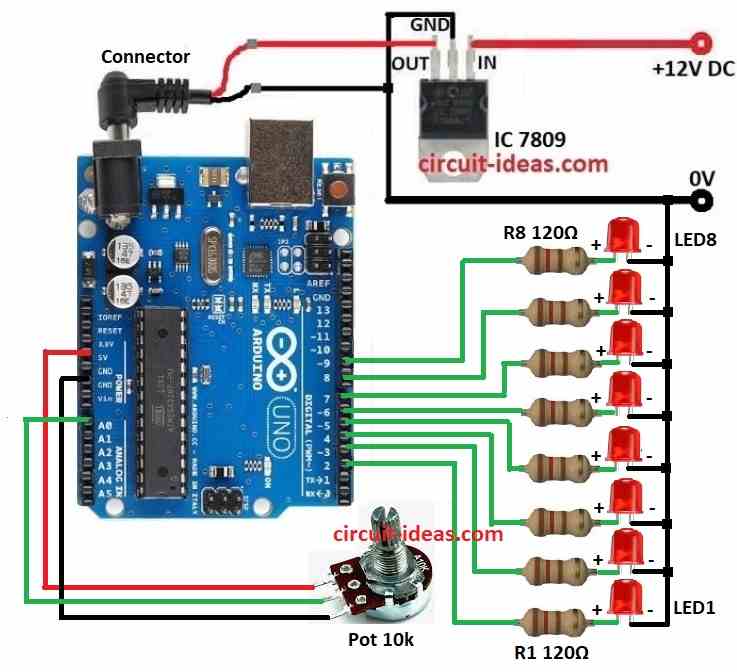
You can arrange the above LEDs in circle as shown below:
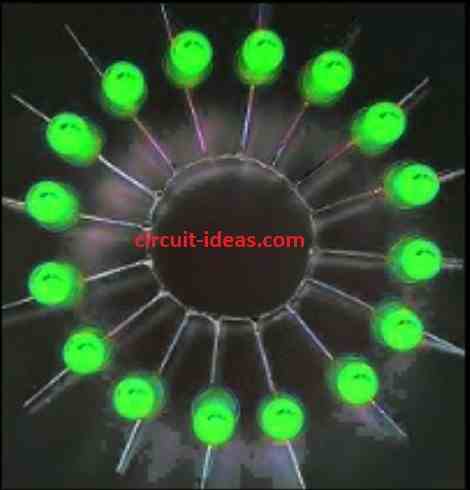
Parts List:
Component | Quantity |
---|---|
Arduino UNO Board | 1 |
IC 7809 Voltage Regulator | 1 |
10k Potentiometer | 1 |
Resistors (1/4 watt, 120Ω) | 8 |
LEDs Red (any color) | 8 |
This article connects the IC 7809s input to the Arduinos 5V and its output to the breadboard power rail for LEDs, making sure the ground pins are connected correctly.
Because it just requires a few external components, building this LED chaser Arduino is quite simple.
We have used a 10k potentiometer to adjust the LEDs flashing and running speeds.
The Arduino Analog pin A0 is linked to the variable end of this resistor as an input.
The potentiometers value is read by the Arduino when the circuit is switched on, adjusting the interval between LED activations.
The pace at which the LEDs are operating will change as you crank the potentiometer.
An eye-catching visual effect will be produced when the LEDs gradually light up in a circular pattern.
Basic principles in programming, electronics, and circuit assembly are introduced through this basic yet effective usage of LEDs.
How to Build:
To build a Simple Arduino-Powered Running LED Circle Circuit follow the below mentioned connections steps:
- Gather all the components as mentioned in the above circuit diagram.
- Connect a regulated IC 7809 to provide a regulated 9V DC to the Arduino board as per the above circuit diagram
- Place 8 LEDs (LED1 to LED8) in a circular pattern on the breadboard.
- Connect a 120Ω resistor (R1 to R8) to the anode legs of each LED.
- Connect a 120Ω resistor (R1 to R8) cathode legs to the ground.
- Connect the other side of each resistor to the following digital pins on the Arduino: 2, 3, 4, 5, 6, 7, 8, and 9.
- Connect the middle pin of the potentiometer to the analog pin A0 on the Arduino.
- Connect one outer pin to 5V and the other to GND as shown in circuit diagram
Conclusion:
An excellent introduction to fundamental electronics and Arduino programming is provided by the Circle Running LED project.
This project shows how to make readily expandable and modifiable interactive displays using standard components like potentiometers, voltage regulators, and LEDs.
This project offers an enjoyable and instructive introduction to the world of electronics, regardless of your level of expertise or need to brush up on your knowledge.
References:
How to control two RGB LED Neopixel Rings (16x pixels each) with Arduino UNO
Leave a Reply