An enjoyable and instructive project that teaches you fundamental electronics, programming and sensor integration in making a water level sensor with an Arduino Uno.
This tutorial will show you how to build a working water level sensor using the widely used Arduino platform, whether your goal is to avoid overflow, monitor water levels in tanks, or establish automatic irrigation systems.
The Arduino Uno microcontroller, renowned for its adaptability and user friendliness, will be used in this project along with a water level sensor module, an IC 7809 voltage regulator, an LED and a resistor.
You may develop and upload code to the Arduino, connect these parts, and analyze sensor data to make decisions depending on water levels by following this guide.
Coding and Explanation:
const int waterSensorPin = 2; // Replace 2 with the actual pin number used for the water level sensor
void setup() {
pinMode(waterSensorPin, INPUT);
Serial.begin(9600);
}
void loop() {
int sensorValue = digitalRead(waterSensorPin);
if (sensorValue == HIGH) {
Serial.println("Water detected");
} else {
Serial.println("No water detected");
}
delay(1000); // Delay for 1 second
}
Explanation:
- The pin number linked to the water level sensor is represented by the water Sensor Pin variable.
- The water Sensor Pin is set up as an input pin and serial connection is started at a baud rate of 9600 in the setup() method.
- The digital Read() method is used in the loop() function to read the value from the water Sensor Pin.
- A message is written to the serial monitor if the value is HIGH, which indicates that water has been detected by the water level sensor.
- In the event that the result is LOW, no water has been found.
- To avoid an overload of messages on the serial display, a one second delay is provided.
Circuit Working:
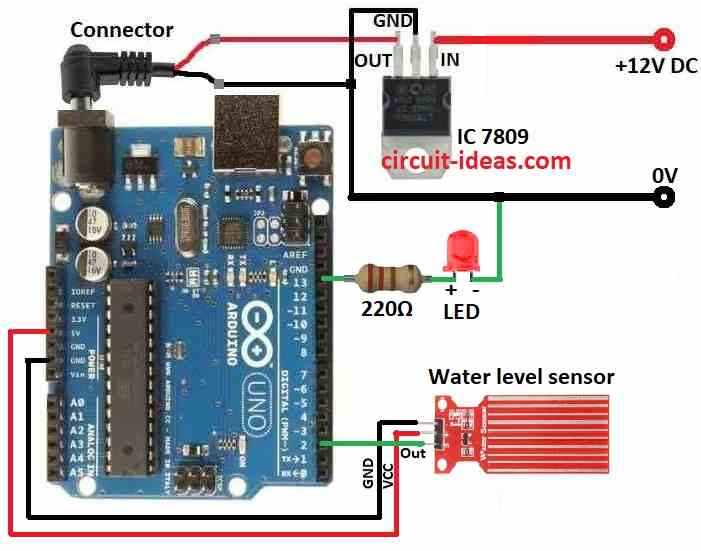
Parts List:
Component | Quantity |
---|---|
Arduino UNO board | 1 |
IC 7809 | 1 |
Water level sensor | 1 |
Resistor 220Ω 1/4 Watt | 1 |
Red LED 5mm 20mA | 1 |
Multimeter | 1 |
Probes Red and Black | 2 |
In the above circuit diagram when the voltage on the output pin increases along with the water level as a drop of water falls inside the glass.
The water detecting component of the sensor on the PCB is what causes this change in voltage output.
The module for measuring water level is made up of two probes.
One of these probes completes a circuit and permits electricity to flow when it is submerged in water.
The other probe serves as a reference point and stays dry.
IC 7809 the Arduino and the water level sensor are powered by a consistent 9V supply from this voltage regulator.
The three pins on the sensor module two of which are power pins need to be linked to the Arduinos ground and 5V pins.
This is necessary for the circuit to operate dependably.
LED and Resistor are to show whether or not there is water present, an LED and resistor are linked in series.
The LED will turn on when the water level sensor finds water.
To keep the LED from burning out, the resistor restricts the amount of current that passes through it.
How to Build:
To build a Water Level Sensor Circuit with Arduino following steps should be followed for connections:
- Gather all the components as mentioned in the above circuit diagram
- Connect a regulated IC 7809 to provide a regulated 9V DC to the Arduino board.
- Connect water level sensor ground pin to ground of Arduino board, VCC pin to 5V pin on Arduino board, and Output pin to pin 2 on Arduino board.
- Connect from pin 13 on Arduino board 220Ω resistor and a Red LED in series and cathode leg of LED to ground pin on Arduino board.
Testing:
- Take a multimeter a device for measuring resistance or voltage.
- Place a tap or container that mimics the appearance of water.
- Turn on the Arduino by connecting it to your PC and turning it on.
- Set up multimeter and select DC voltage measurement on the multimeter.
- Now attach the multimeters positive probe to the water level sensors OUT pin.
- And set the multimeters negative probe against the sensors GND pin.
- When the sensor is dry, look at the voltage measurement on the multimeter.
- The voltage should be high or low based on the output properties of the sensor.
- Check that the voltage reading matches the desired state e.g. HIGH for water detected, LOW for no water according to your Arduino code.
- Slowly immerse the sensor in water, then use the multimeter to detect the voltage.
- The voltage should change to the opposite state as soon as the sensor comes into contact with water.
- Make sure the voltage change corresponds to what your Arduino code expects it to.
- To guarantee consistent and trustworthy readings, repeat steps 4 and 5 several times.
Conclusion:
Using the above components mentioned in circuit diagram you may construct a working circuit that reliably detects the presence or absence of water by following the instructions provided.
More sophisticated functionality, such issuing alarms or managing other devices based on the water level, might be added to this project.
Leave a Reply