An inverter is a really important electronic gadget that changes DC Direct Current power into AC Alternating Current power.
In this project, we are going to create a basic inverter circuit powered by an Arduino that can turn a 12V DC supply into 230V AC.
We will use an Arduino UNO, IC 7809, some transistors and a transformer that works in reverse to get the AC output.
This kind of circuit can come in handy for running small devices when the power goes out.
Circuit Working:
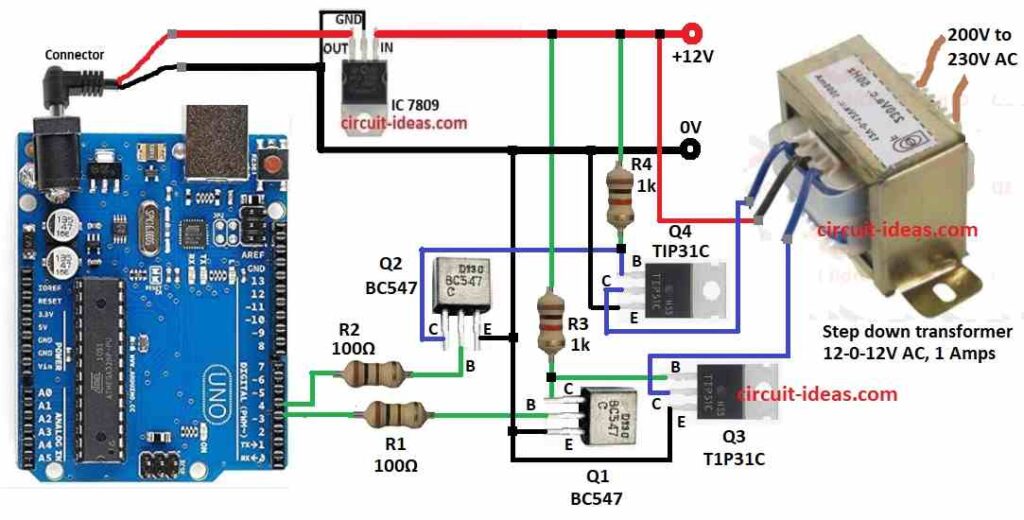
Parts List:
Component | Quantity |
---|---|
Arduino UNO Board | 1 |
Resistors 100Ω, 1k (1/4 watt) | 2 each |
IC 7809 | 1 |
Transistors BC547, TIP31C | 2 each |
Step down transformer 12-0-12V AC, 1 Amps | 1 |
The IC 7809 makes sure that the Arduino UNO and the control circuit get a steady 9V power supply.
This helps the circuit work more reliably.
In this article the Arduino UNO sends out square wave signals from pins D3 and D4.
These signals work in opposition so when one pin is HIGH the other is LOW.
This back and forth creates the pulses needed to operate the transistors.
The BC547 transistors Q1 and Q2 serve as amplifiers for these signals.
They take the low current signals from the Arduino and boost them to control the base terminals of the TIP31C power transistors Q3 and Q4.
Resistors R1 and R2 which are 100Ω each help limit the base current to keep the BC547 transistors safe.
The TIP31C transistors Q3 and Q4 act as high current switches taking turns to power the primary winding of the step down transformer.
Resistors R3 and R4, each 1k help ensure that the TIP31C transistors are properly biased.
The step down transformer is set up in reverse.
When it receives the alternating signals from the TIP31C transistors, it increases the 12V DC to about 230V AC at its secondary winding.
Coding with Explanation
const int pin1 = 3; // Pin D3 connected to Q1
const int pin2 = 4; // Pin D4 connected to Q2
void setup() {
pinMode(pin1, OUTPUT);
pinMode(pin2, OUTPUT);
}
void loop() {
digitalWrite(pin1, HIGH);
digitalWrite(pin2, LOW);
delay(10); // 50Hz frequency (20ms period, 10ms HIGH per side)
digitalWrite(pin1, LOW);
digitalWrite(pin2, HIGH);
delay(10);
}
Code Explanation:
- The code makes pins D3 and D4 work as outputs.
- Inside the loop, the Arduino switches D3 to HIGH and D4 to LOW, then flips them after a 10ms pause.
- This action produces a square wave with a frequency of 50Hz to control the transistors.
Formulas:
Below mentioned is the formulas for frequency calculations for Arduino Based Power Inverter Circuit:
Frequency (ƒ) = 1 / Period
- The total period is 20ms (10ms HIGH + 10ms LOW for each signal).
- ƒ = 1 / 0.02s = 50Hz.
Cautions:
- Make sure to use a good heat sink for the TIP31C transistors because they deal with a lot of power.
- It is also important to include a fuse or some kind of overcurrent protection to keep the circuit safe.
- Always test the circuit with someone watching, since the high voltage coming from the transformer can be dangerous.
How to Build:
To build a Arduino Based Power Inverter Circuit follow the below mentioned steps for connections:
Gather all the components as mentioned in the above circuit diagram
- Connect the 12V DC power supply to the input pin of the IC 7809.
- Connect the ground pin of the IC 7809 to the GND of the circuit.
- The output pin of the IC 7809 provides a stable 9V supply, which is connected to the VIN pin of the Arduino UNO board.
- Connect digital pin D3 to the base of the BC547 transistor Q1 through a 100Ω resistor R1
- Connect digital pin D4 to the base of the BC547 transistor Q2 through another 100Ω resistor R2
- Connect the emitters of both BC547 transistors Q1 and Q2 to the ground.
- Connect the collectors of Q1 and Q2 to the base pins of TIP31C transistors Q3 and Q4 through 1k resistors R3 and R4, respectively.
- Connect the emitters of the TIP31C transistors Q3 and Q4 to the ground.
- The collectors of Q3 and Q4 are connected to opposite ends of the primary winding of the step down transformer.
- Use a step down transformer 12V to 230V connected in reverse.
- The center tap of the primary winding is connected to the 12V power supply.
- The secondary winding of the transformer outputs the 230V AC.
Conclusion:
This easy Arduino Based Power Inverter Circuit shows how to create AC power from a DC source using simple parts.
Although this setup is good for low power uses, it helps you learn how inverters work and can be changed to improve performance or handle more power.
Leave a Reply